The Singapore Land Authority (SLA) launched an alternative to Google Maps recently - called OneMap. It's built on ESRI's ArcGIS server and it comes with its OneMap Javascript API, which is essentially a wrapper around ESRI's API. This gives me a chance to work with ESRI's Javascript API and I decided to port the Charting Google Mapplet I wrote earlier to OneMap. The figure below is a screen shot of the gadget running in iGoogle's expanded Canvas mode.
This gadget will create pie charts on Singapore's OneMap from text files formatted as comma separated values (CSV). The CSV data must have a header row, SVY21 easting and northing columns to point to the locations to place the charts on. The charts created can be clicked on to show the values.
For IE8 users, please run in IE7 or IE8 compatibility mode as IE8 breaks OneMap.The figure below shows the problem of OneMap with Internet Explorer 8 on iGoogle. I am not able to determine the source of the problem but you can click IE's Page button and choose Compatibility View Settings to turn add google.com to the Compatibility View websties .
To run this gadget, click this link http://www.google.com/ig/adde?moduleurl=http://dominoc925-pages.appspot.com/gadgets/chart_onemap_ig.xml&source=imag.
Friday, April 30, 2010
Monday, April 26, 2010
Now GeoMedia can read ArcView Projection Files (*.prj)
The recent GeoMedia hot fix 6.1.7.16 added a new function in the Define Coordinate System File utility to load ArcView coordinate projection files (*.prj). Previously, you could only read in coordinate systems from Microstation type 56 elements in design files (*.dgn) and other GeoMedia coordinate system files (*.csf). Reading in the prj file is similar to reading in the dgn and csf files as shown below.
- Click Start | All Program | GeoMedia Professional | Utilities | Define Coordinate System File.
The Define Coordinate System File dialog appears.
- Click Load.
The Load Coordinate System From File dialog appears.
- Click the Files of Type combo box. Choose Projection Files (*.prj). Choose a *.prj file on your system. Click Open.
The coordinate system is read.
Friday, April 23, 2010
Google Mapplet for creating Piecharts
Creating charts on maps have been available on desktop mapping software such as MapInfo for many years but not for GeoMedia, though there was some an add-on GeoMedia Business Analysis software from Europe several years ago.I thought this would be an interesting thing to do on the Internet ala Cloud Computing. After reading (skimming) through the Google Maps API and Google Visualization API, I thought that it was possible to do and so I set out to write a Charting Mapplet in my free time. I have completed it as can be seen in the screen shot below.
This Mapplet will create pie charts on Google Maps from text files formatted as comma separated values (CSV). The CSV data must have a header row, geographic latitude and longitude columns to point to the locations to place the charts on. The charts created can be clicked on to show the values. The charts can also be dragged and moved to a different location on the map if necessary.
If you want to take this for a spin, click on this link http://dominoc925-pages.appspot.com/mapplets/piechart.html.
This Mapplet will create pie charts on Google Maps from text files formatted as comma separated values (CSV). The CSV data must have a header row, geographic latitude and longitude columns to point to the locations to place the charts on. The charts created can be clicked on to show the values. The charts can also be dragged and moved to a different location on the map if necessary.
If you want to take this for a spin, click on this link http://dominoc925-pages.appspot.com/mapplets/piechart.html.
Thursday, April 15, 2010
Google Gadget for SVY21 Coordinates Conversion Calculator
Note: A WebApp version of this Google Gadget is available here http://dominoc925-pages.appspot.com/webapp/calc_svy21/default.html.
SVY21 is a Transverse Mercator projection (with a WGS84 datum) commonly used in Singapore. This Google Gadget will allow you to convert between one or more pairs of SVY21 easting, northing coordinates and geographical latitude, longitude coordinates. The gadget can be embedded to your iGoogle home page or your web page.
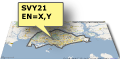
Wednesday, April 7, 2010
How to create custom Google Maps Marker Tool Tips
The default Google Maps markers have mouse over tool tips but with the standard light yellow box. With some relatively simple Javascript code and cascading style sheet declarations, you can quickly create your own marker tool tips with a custom style. The basic steps are:
As shown below in the sample html code, define a style class (e.g. toolTip) for the tool tip so that the tool tip has a light yellow background with small text and a solid dark gray border.
Create the Tool TIp Child DIV Element
Traditionally, a Google Maps instance is placed on a web page through a DIV element in the HTML code. In this example, that element has an ID of "map". To show tool tips on this Google Maps instance, we need to create a child DIV element underneath it using Javascript.
Create the markers
Now create the markers and add them as overlays on the map. If you are using custom icons, the clickable property must be set to be true in order to trigger the mouse events.
Create and register the mouse event handlers
To show the tool tips as the mouse move over the markers, create and register the showToolTip function. Basically, this function simply shows the child tool tip DIV element. Also create and register the hideToolTip function, which just hides the child tool tip DIV element.
That's all there is to it.
- Declare a tool tip style.
- Create a child DIV element for the tool tip under the parent Google Maps DIV element.
- Create the markers and set the tool tip text property
- Show the tool tip DIV element when the mouse hovers over the marker
- Hide the tool tip DIV element when the mouse exits the marker
As shown below in the sample html code, define a style class (e.g. toolTip) for the tool tip so that the tool tip has a light yellow background with small text and a solid dark gray border.
<html>
<head>
<title>My first tool tip</title>
<style type="text/css">
.toolTip {
font-size: small;
background-color:#ffff71;
border:1px #7f7f7f solid;
}
</style>
</head>
<body>
<div id="map" style="height: 450px;" >
Loading...
</div>
</body>
</html>
Traditionally, a Google Maps instance is placed on a web page through a DIV element in the HTML code. In this example, that element has an ID of "map". To show tool tips on this Google Maps instance, we need to create a child DIV element underneath it using Javascript.
var map;
var toolTip;
var divToolTip = "toolTip";
var divMapId = "map";
var icon = new GIcon(G_DEFAULT_ICON);
var pnt = new GLatLng(1.3629,103.8263);
//Create a new Google Maps instance
map = new GMap2(document.getElementById(divMapId));
//create the child tool tip DIV element and hide it
toolTip = document.createElement(divToolTip);
document.getElementById(divMapId).appendChild(toolTip);
toolTip.style.visibility="hidden";
Create the markers
Now create the markers and add them as overlays on the map. If you are using custom icons, the clickable property must be set to be true in order to trigger the mouse events.
//Create the marker
var opt = {};
opt.icon = icon;
opt.draggable = false;
//clickable must be set to true to trigger the mouse events
opt.clickable = true;
opt.dragCrossMove = false;
var mkr = new GMarker (pnt, opt);
mkr.tooltip='<div class="toolTip">'+ 'My first tooltip' + '<\/div>';
map.addOverlay(mkr);
Create and register the mouse event handlers
To show the tool tips as the mouse move over the markers, create and register the showToolTip function. Basically, this function simply shows the child tool tip DIV element. Also create and register the hideToolTip function, which just hides the child tool tip DIV element.
//Register the marker event handlers
GEvent.addListener(mkr,"mouseover", function() {
showToolTip(mkr);
});
GEvent.addListener(mkr,"mouseout", function() {
hideToolTip();
});
//function to hide the tool tip
function hideToolTip () {
toolTip.style.visibility="hidden";
};
//function to show the tool tip
function showToolTip(marker) {
toolTip.innerHTML = marker.tooltip;
var point=map.getCurrentMapType().getProjection().fromLatLngToPixel(map.getBounds().getSouthWest(),map.getZoom());
var offset=map.getCurrentMapType().getProjection().fromLatLngToPixel(marker.getPoint(),map.getZoom());
var anchor=marker.getIcon().iconAnchor;
var width=marker.getIcon().iconSize.width;
var pos = new GControlPosition(G_ANCHOR_BOTTOM_LEFT, new GSize(offset.x - point.x - anchor.x + width,- offset.y + point.y +anchor.y));
pos.apply(toolTip);
toolTip.style.visibility="visible";
};
That's all there is to it.
Thursday, April 1, 2010
Singapore Weather Google Gadget
This embedded Google Gadget shows the current weather in Singapore on a Google Maps backdrop. You can leave this gadget on the screen and it will update itself with the latest weather information from the National Environment Agency (NEA) rss feed. The weather information is displayed as icons which will display tool tips of the location as you hover over the icons.
Subscribe to:
Posts (Atom)